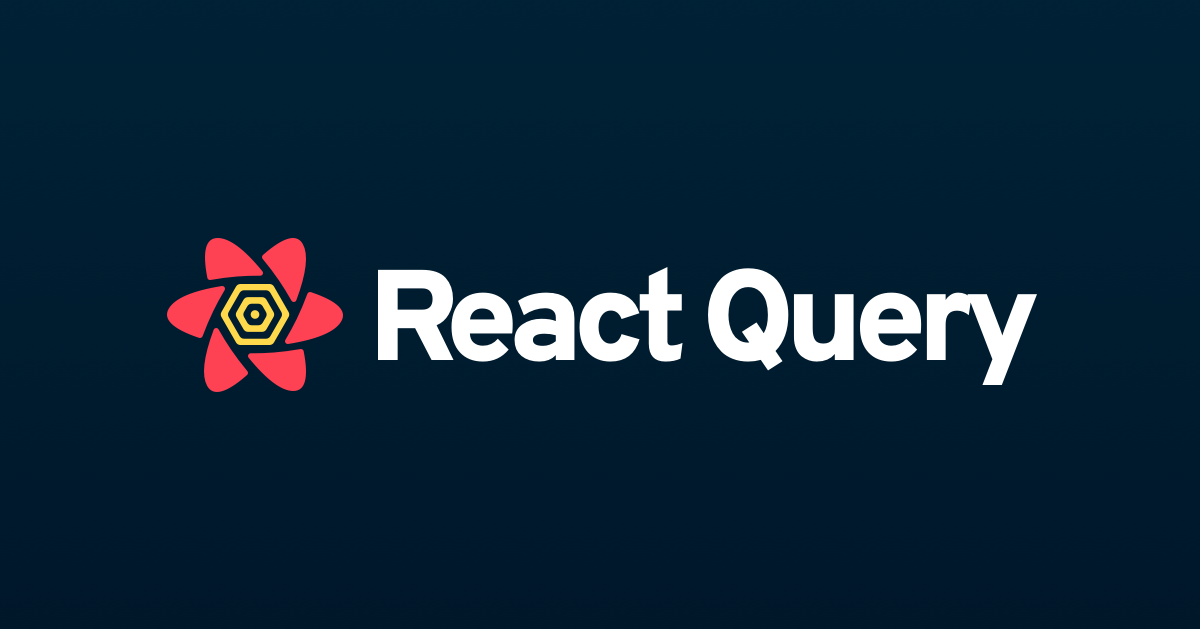
What is useQuery?
The useQuery hook is the primary API for executing queries in a React application. We run a query within a React component by calling useQuery and passing it our GraphQL query string. This makes running queries from React components a breeze.
Is useQuery a hook?
useQuery is a hook that makes the GraphQL query a side-effect and returns data, loading, and error containing the GraphQL response, the request state, and the request error respectively.
Does it useQuery cache?
By default, the useQuery hook checks the Apollo Client cache to see if all the data you requested is already available locally. If all data is available locally, useQuery returns that data and doesn’t query your GraphQL server.
Is useQuery asynchronous?
The useQuery hook is a function used to register your data fetching code into React Query library. It takes an arbitrary key and an asynchronous function for fetching data and returns various values that you can use to inform your users about the current application state.
Does useQuery run before useEffect?
It doesn’t have to be before useQuery, though, just somewhere before the if statements. This does mean that the hook may be executed before the query data is available, but that is fine – you’ll just have to handle these cases inside your useEffect hook, as you already seem to be doing, considering your data check.
Does useQuery run on every render?
The useQuery hook will make one fetch request on the initial load, and will not refetch on subsequent renders. The useQuerey hook will trigger a re-render when it receives a response from the graphql backend (whether that response is data or an error ).
What is a lazy query?
Lazy query: Lazy queries keep a pointer to the database and only load the data on demand. If you loop through a query the data is loaded on the spot. It does not create a two-dimensional struct to store all the data hand. When the query tag is done, it keeps a pointer to the database.
Syntax:
const {
2 data,
3 dataUpdatedAt,
4 error,
5 errorUpdatedAt,
6 failureCount,
7 isError,
8 isFetched,
9 isFetchedAfterMount,
10 isFetching,
11 isIdle,
12 isLoading,
13 isLoadingError,
14 isPlaceholderData,
15 isPreviousData,
16 isRefetchError,
17 isRefetching,
18 isStale,
19 isSuccess,
20 refetch,
21 remove,
22 status,
23 } = useQuery(queryKey, queryFn?, {
24 cacheTime,
25 enabled,
26 initialData,
27 initialDataUpdatedAt
28 isDataEqual,
29 keepPreviousData,
30 meta,
31 notifyOnChangeProps,
32 notifyOnChangePropsExclusions,
33 onError,
34 onSettled,
35 onSuccess,
36 placeholderData,
37 queryKeyHashFn,
38 refetchInterval,
39 refetchIntervalInBackground,
40 refetchOnMount,
41 refetchOnReconnect,
42 refetchOnWindowFocus,
43 retry,
44 retryOnMount,
45 retryDelay,
46 select,
47 staleTime,
48 structuralSharing,
49 suspense,
50 useErrorBoundary,
51 })
or using the object syntax:
const result = useQuery({
queryKey,
queryFn,
enabled,
})
Happy Coding!