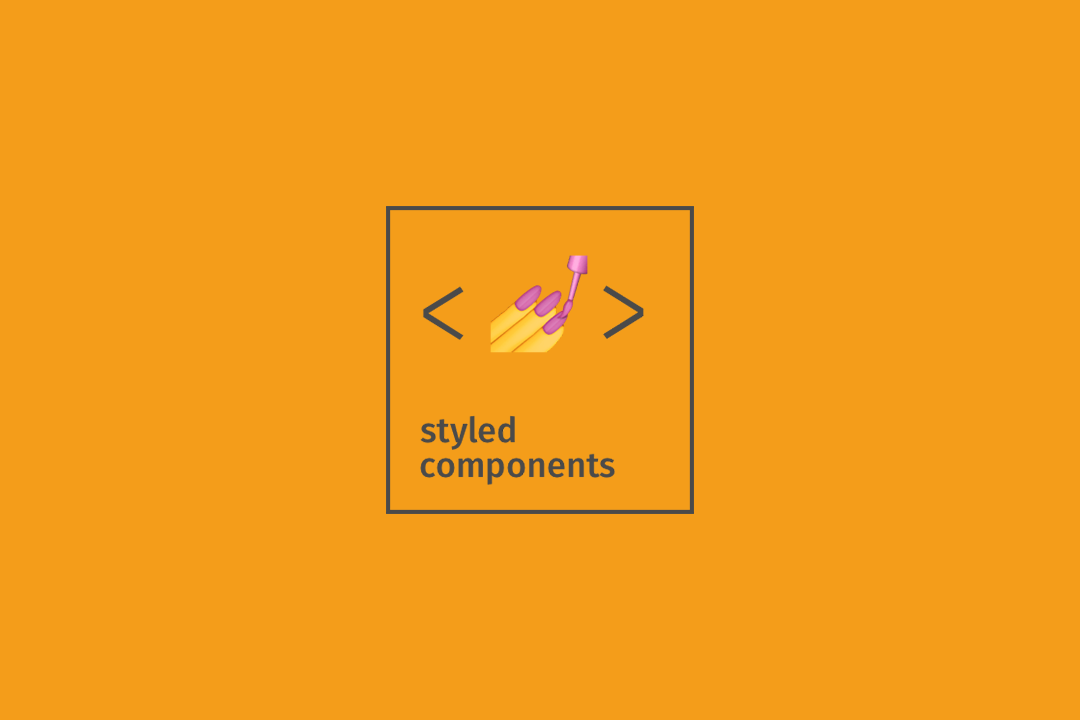
Styled components are react components with styles. Styled-components make it simple to use CSS by writing JavaScript code. Well, why do we use styled components instead of CSS modules? The answer is pretty simple, styled components provide us benefits some of them are described below;
- Styled components apply on those components which are wrapped in.
- It automatically cleans up unused CSS declarations which reduces the bundle size.
- DOM elements are assigned with unique class names, to avoid clashes and bugs, styled components allow us to build our app in a stress less manner.
- Styled components allow us to dynamically style our components with props.
- Maintenance becomes easy no matter how large your code is, because you do not need to discover all the files that affect your component.
Let’s get started!
First we need to create react app using command
npx create-react-app styled-components-demo
Then install a package using npm or yarn.
# with npm
npm install –save styled-components
#with yarn
yarn add styled-components
Now we are ready to go!
In /src/app.js create a styled button component and use it in the render method.
import styled from "styled-components";
const Button = styled.button`
color: white;
background-color: #2192ff;
padding: 10px 15px;
border-radius: 5px;
border: none;
margin-right: 5px;
`;
function App() {
return (
<div className="App">
<Button>Normal Button</Button>
</div>
);
}
export default App;
Output

What if we want to create a button with a different background colour, do we need to create another button component? No it’s not, we just need to pass props in the Button component for dynamic styling.
import "./App.css";
import styled from "styled-components";
const Button = styled.button`
color: white;
background-color: ${(props) =>
props.success ? "#38E54D" : props.danger ? "#DC3535" : "#2192ff"};
padding: 10px 15px;
border-radius: 5px;
border: none;
margin-right: 5px;
`;
function App() {
return (
<div className="App">
<Button>Normal Button</Button>
<Button success>Success</Button>
<Button danger>Danger</Button>
</div>
);
}
export default App;
Output
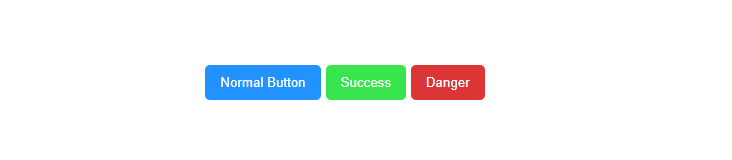
There are several other functions which we also use like to reverse the text of the button
import "./App.css";
import styled from "styled-components";
const Button = styled.button`
color: white;
background-color: ${(props) =>
props.success ? "#38E54D" : props.danger ? "#DC3535" : "#2192ff"};
padding: 10px 15px;
border-radius: 5px;
border: none;
margin-right: 5px;
`;
const ReversedButton = (props) => (
<Button {...props} children={props.children.split("").reverse()} />
);
function App() {
return (
<div className="App">
<Button>Normal Button</Button>
<Button success>Success</Button>
<Button danger>Danger</Button>
<Button as={ReversedButton}>Reversed Text</Button>
</div>
);
}
export default App;
Output
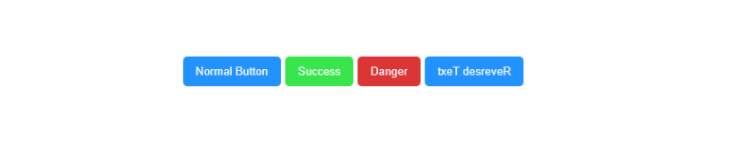
You might be thinking, where do I declare my global styles? Here creatingGlobalStyle comes into picture.
Global Styles
Normally the styles components are scoped to particular components but in creatingGlobalStyle the limitations regarding scope are not counted.
Create a Global.js file and a component which has global styles.
import { createGlobalStyle } from "styled-components";
export const GlobalStyles = createGlobalStyle`
* {
box-sizing: border-box;
padding: 0;
margin: 0;
}
body {
color: black;
background: gray;
}
`;
Add <GlobalStyles /> in app.js
import "./App.css";
import styled from "styled-components";
import { GlobalStyles } from "./Global";
const Button = styled.button`
color: white;
background-color: ${(props) =>
props.success ? "#38E54D" : props.danger ? "#DC3535" : "#2192ff"};
padding: 10px 15px;
border-radius: 5px;
border: none;
margin-right: 5px;
`;
const ReversedButton = (props) => (
<Button {...props} children={props.children.split("").reverse()} />
);
function App() {
return (
<div className="App">
<GlobalStyles />
<Button>Normal Button</Button>
<Button success>Success</Button>
<Button danger>Danger</Button>
<Button as={ReversedButton}>Reversed Text</Button>
</div>
);
}
export default App;
Output

As you saw the background color is now gray.
In summary, regardless of the situation, it’s bad practice to save all of your styles in one file as it defeats the essence of modularization and component-based styling features that styled-components provides.